Exercise 1: Display a list of items with a ListView (XAM280)
In this exerise, you will add a ListView to an existing application to display a data collection.
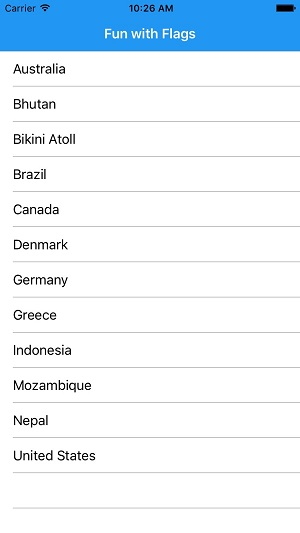
To complete the exercise, you will need Visual Studio for Windows or macOS with the Xamarin development tools installed. You will also need either an emulator/simulator or a device to run the exercise on. Please see the setup page if you need help installing the Xamarin development environment.
Open the starter solution
- Open the starter solution from the Exercise 1 > Start folder in your copy of the cloned or downloaded course materials in either Visual Studio on Windows or Visual Studio for Mac.
- Build and run the application. The app displays a single flag, allows you to edit the details of the flag, and lets you switch between the flags using toolbar buttons.
- The solution contains several projects. The shared Xamarin.Forms UI is defined in the FunFlacts Portable Class Library (PCL), and there are head projects for iOS, Android and Windows. There is also a FlagData project that contains the flag information.
- Examine the classes you'll work with:
File | Description |
---|---|
App.cs | Contains the Application class for our Xamarin.Forms application which defines the starting page for the application; this is currently the FlagDetailsPage . |
FlagDetailsPage.xaml(.cs) | Defines the UI and logic for our initial page. |
FunFlactsViewModel.cs | This is in the FlagData project and contains the flag sample data. It exposes a collection property (Flags ) which returns a IList<Flag> . A single instance of this object is registered with the Xamarin.Forms DependencyService . |
Flag.cs | This defines the data for a single flag. It implements INotifyPropertyChanged and provides several details about the flag. |
Create a XAML content page
Replace the current startup page with a new ContentPage
.
- Add a new XAML
ContentPage
to the FunFlacts project. Name it AllFlags. - Open the AllFlags.xaml file and set the
Title
property to "Fun with Flags". - Delete any existing UI from the XAML.
- Open the App.xaml.cs file and go to the constructor.
- Create an instance of your AllFlags content page.
- Create a new
NavigationPage
(or reuse the existing code), passing in the AllFlags instance. - Assign the
NavigationPage
(containing the AllFlags page) to theMainPage
property. -
Build and run the app. It will display an empty page. You'll add a
ListView
to the page in the following steps.public App() { DependencyService.Register<FunFlactsViewModel>(); InitializeComponent(); MainPage = new NavigationPage(new AllFlags()); }
Add a ListView to the page
The ListView needs to be populated with data. There's a collection of Flag data defined in FunFlactsViewModel
that you'll load into the ListView.
- Open AllFlags.xaml.
-
Add a
ListView
as the root tag in theContentPage
.<?xml version="1.0" encoding="utf-8" ?> <ContentPage xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" Title="Fun with Flags" x:Class="FunFlacts.AllFlags"> <ListView> </ListView> </ContentPage>
-
Load the
FunFlactsViewModel.Flags
into to theListView.ItemsSource
property. There are several ways to do this, there are examples below:- Give the
ListView
a name flags in the XAML and set theItemsSource
property in code behind. You can retrieve theFunFlagsViewModel
instance using theDependencyService.Get<FunFlactsViewModel>()
method.
flags.ItemsSource = DependencyService.Get<FunFlactsViewModel>().Flags;
- Give the
-
Set the
BindingContext
for theContentPage
to theFunFlactsViewModel
instance in code behind then use a{Binding}
on theListView.ItemsSource
property to connect it to theFlags
property.BindingContext = DependencyService.Get<FunFlactsViewModel>();
<ListView ItemsSource="{Binding Flags}" />
-
Run the application, it should now display a list of items. Notice that it's displaying the type name (
FlagData.Flag
) for each item. This is because theListView
uses theToString()
method by default. -
Open the Flag.cs file in the FlagData project and
override
theToString
method. Have the method return theCountry
property. -
Run the application to the see the effect.
Exercise summary
In this exercise, you learned how to display a collection in Xamarin.Forms. This is a fundamental operation that you'll likely use in most applications that you build.
You can view the completed solution in the Exercise 1 > Completed folder of your copy of the cloned or downloaded course materials.