Exercise 2: Use StackLayout to build a UI
The goal of this lab is to use nested StackLayout
s to arrange the views in a UI.
The first screenshot below shows the Starter project and the second shows the Completed project. Your job is to use StackLayout
s and LayoutOptions
to turn the starter project into the completed version. There is no single "correct" answer here. Use your judgment to produce a UI that looks good to you.
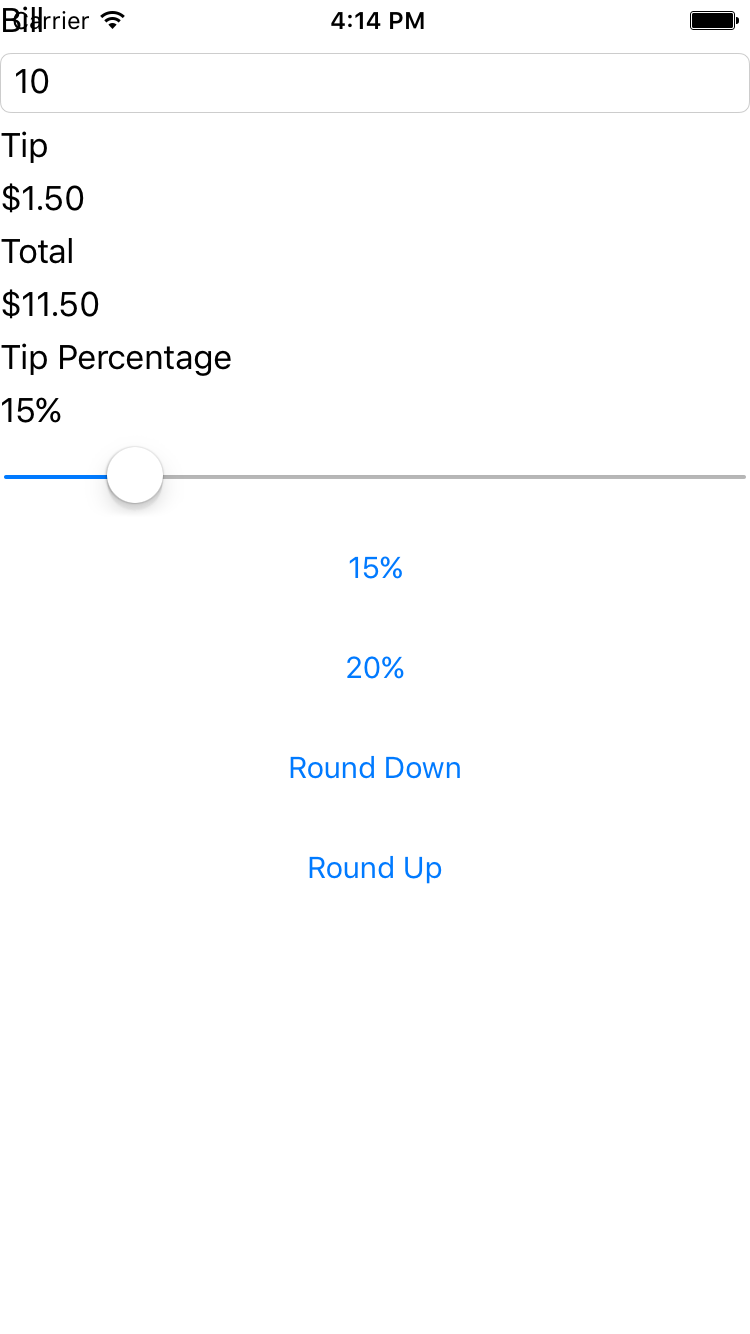
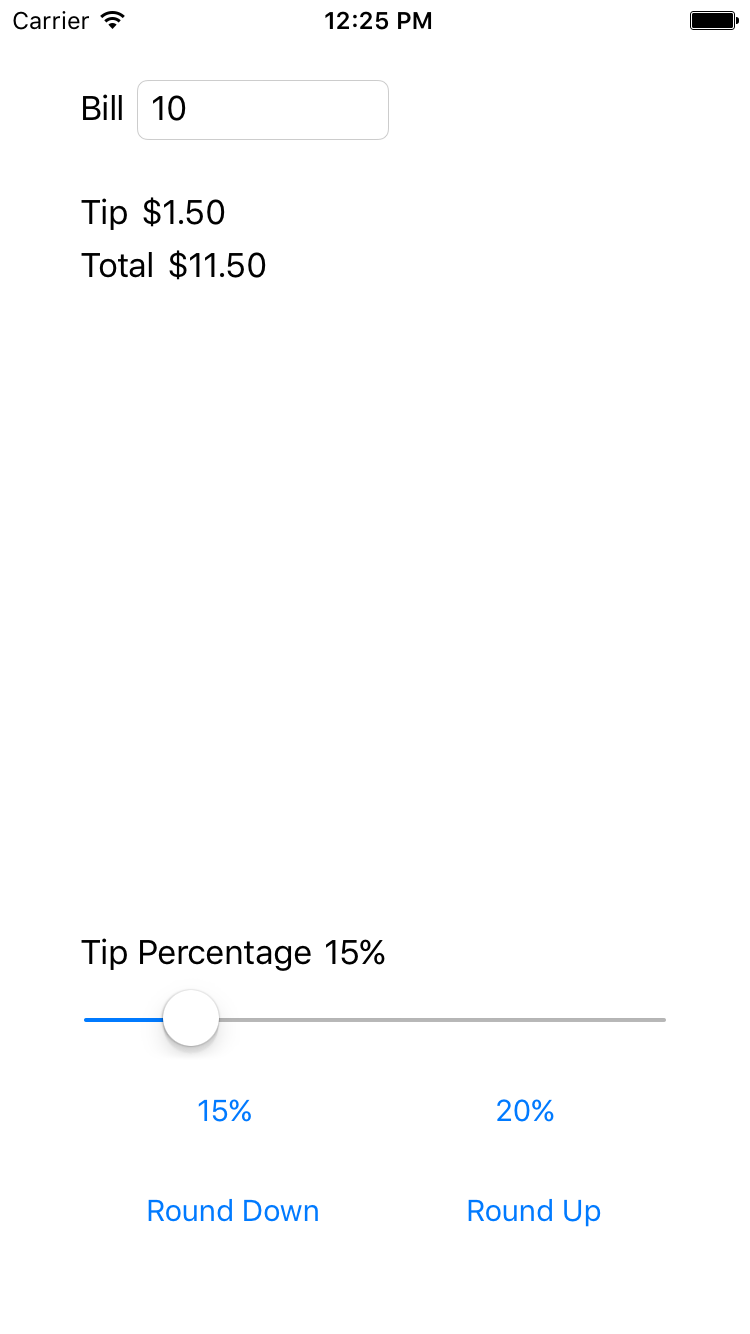
The images shown above may be sufficient to allow you to complete the lab. If you would like addition guidance, please use the steps on the next pages.
To complete the exercise, you will need Visual Studio for Windows or macOS with the Xamarin development tools installed. You will also need either an emulator/simulator or a device to run the exercise on. Please see the setup page if you need help installing the Xamarin development environment.
Explore the starter code
The starter solution contains a fully functional TipCalculator app. Your goal in this first part is to explore how the app works.
- Open the Exercise 2 > Start solution from your copy of the cloned or downloaded course materials in either Visual Studio for Windows or Visual Studio for Mac.
- Run the app on a platform of your choice.
- Enter a number into the
Entry
control - the app should then look similar to the image shown below:
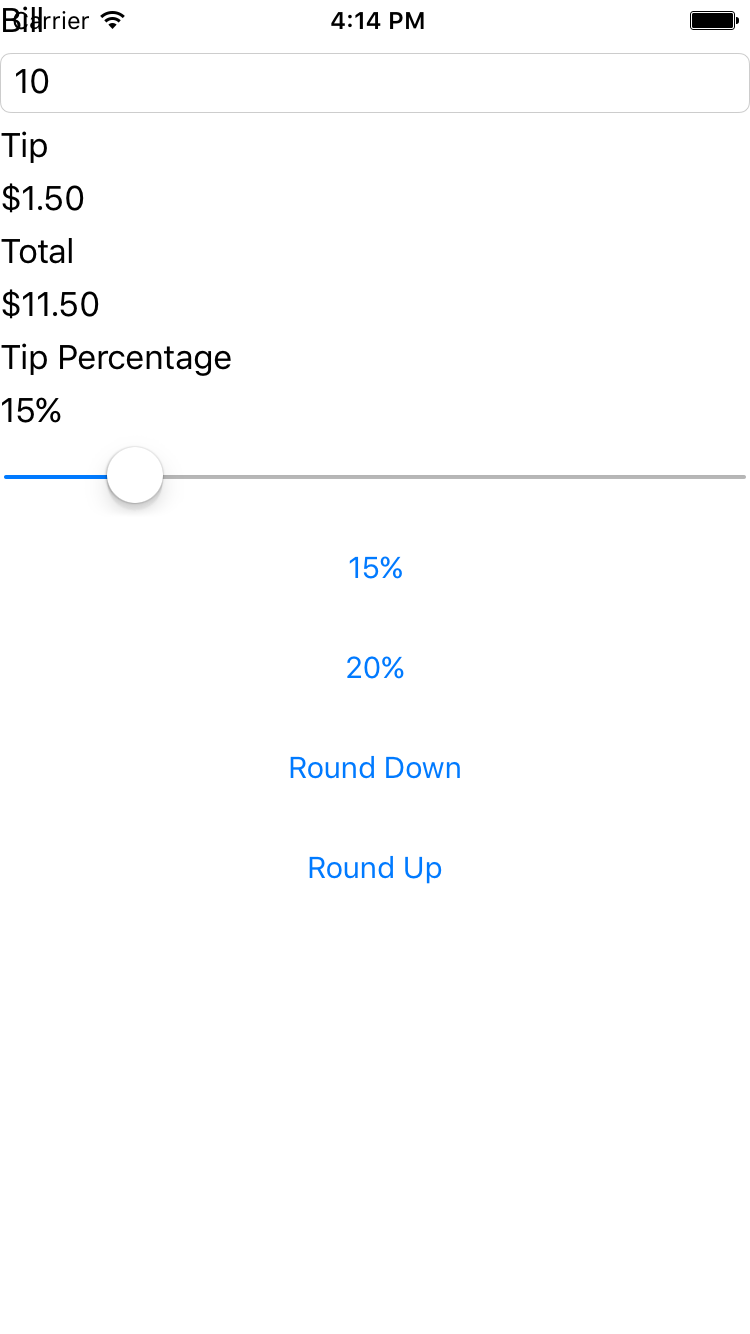
- Work with the app for a minute or two to get a feel for its behavior.
- Stop the app.
- Open MainPage.xaml.
- Notice that all the views are placed into one vertical
StackLayout
. - If you would like to, feel free to open MainPage.xaml.cs and look at the code; however, you will not need to modify the code-behind during the exercise.
Detailed instructions
This section gives step-by-step guidance to layout the TipCalculator UI as it was pictured in the Completed screenshot. Attempt to create the UI yourself before following these steps. If you have already finished the exercise based on the high-level goals given earlier, you can either review these instructions or skip to the next section.
Please run the app as needed to test your work as you go through these steps.
- Add
Padding
to the page to avoid overlap of the UI and the iOS status bar. TheTop
value for thePadding
should be at least20
. The code shown below will yield a uniformPadding
of40
units:
<ContentPage ... Padding="40">
- Use a horizontal
StackLayout
to group theLabel
that says "Bill" with theEntry
field as shown below:
<StackLayout Orientation="Horizontal">
<Label Text="Bill" ... />
<Entry ... />
</StackLayout>
- Use a horizontal
StackLayout
to group theLabel
that says "Tip" with theLabel
namedtipOutput
. - Set a
Margin
on theStackLayout
you just added. The top should be 20 units while the left, right, and bottom values should be 0 (see below).
<StackLayout Orientation="Horizontal" Margin="0,20,0,0">
...
</StackLayout>
- Use a horizontal
StackLayout
to group theLabel
that says "Total" with theLabel
namedtotalOutput
. - Use a horizontal
StackLayout
to group theLabel
that says "Tip Percentage" with theLabel
namedtipPercent
. - Set a
VerticalOptions
value ofEndAndExpand
on theStackLayout
you just added (see below).
<StackLayout Orientation="Horizontal" VerticalOptions="EndAndExpand">
...
</StackLayout>
- Use a horizontal
StackLayout
to group theButton
with text of "15%" and the Button with text "20%". - Use a horizontal
StackLayout
to group theButton
with text of "Round Down" and theButton
with text "Round Up". - Set a
HorizontalOptions
value ofCenterAndExpand
on all four of theButtons
(see below).
<Button HorizontalOptions="CenterAndExpand" ...>
Exercise summary
In this exercise, you used nested StackLayout
s to improve the aesthetics of an existing UI. StackLayout
is the simplest layout panel, yet it is powerful enough to produce a reasonable UI.
You can view the completed solution in the Exercise 2 > Completed folder of your copy of the cloned or downloaded course materials.