Duration
10 minutes
Goals
The primary goal of this lab is to modify an existing application to use the task-based async pattern when downloading search results from a web service.
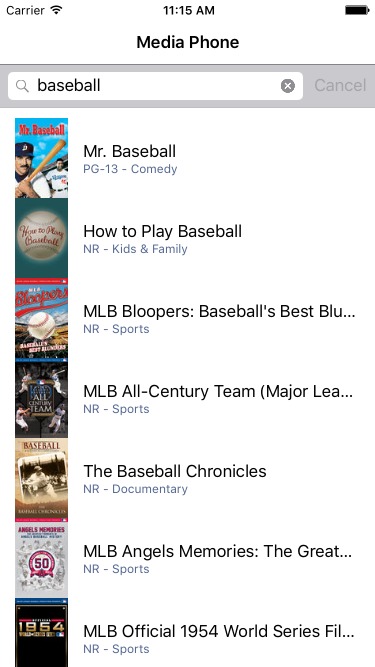
Required assets
This exercise is a continuation from the previous exercise. You may use your existing solution or start with the Completed solution in the Exercise 1 folder. The provided Exercise 2 folder contains a subfolder named Completed with a solution you can use to check your work. Please make sure you have this folder before you begin.
Challenge
Update an existing application to use the task-based async pattern when searching for movies from a web service.
- Open MovieApi.cs and replace the SearchAsync method with a task-based version. Use the synchronous Search method as a starting-point.
- Call the new SearchAsync method from within the OnSearch method in SearchPage.xaml.cs.
Steps
Create a new Async Search Method
- Open MovieApi.cs in the shared project MediaPhone.
- Comment-out or delete the existing SearchAsync method, we're going to replace it.
- Create a new
static
method named SearchAsync, copying the signature from the original Search method. The new method should return anIEnumerable
ofSearchItem
's and accept a single string parameter named text. - Next, copy the contents of the original Search method into your new SearchAsync method.
Modify Search Async to use the Task Async Pattern
- Add the
async
keyword to the method signature before the return type. - Change the return type to return a
Task
that encapsulates theIEnumerable
ofSearchItem
s. - Change the method call on the
WebClient
to download the results to use the new method; change it fromDownloadString
toDownloadStringTaskAysnc
. - Note that
DownloadStringTaskAsync
returns aTask
so we can now use theawait
keyword. Await the call toDownloadStringTaskAsync
.
Call the Task-based Async Search Method
- Open SearchPage.xaml.cs.
- Restore the OnSearch method to its original synchronous form:
void OnSearch (object sender, EventArgs e) { if (string.IsNullOrEmpty(searchBar.Text)) return; Data.Clear(); searchProgress.IsRunning = true; try { var results = MovieApi.Search(searchBar.Text); foreach (var item in results) Data.Add(item); } catch (WebException ex) { DisplayAlert("Error", ex.Message + "\r\nAre you connected to the Internet?", "OK"); } finally { searchProgress.IsRunning = false; } }
- Change the call to the static MovieApi.Search method to MovieApi.SearchAsync.
- Since this method returns a
Task
any calls to this method should be awaited. Place theawait
keyword before the call to SearchAsync. - In order to use the
await
keyword, our method needs to be marked as async. Add theasync
keyword before the method signature of OnSearch. - We can make one additional optimization now that our OnSearch method is asynchronous. Examine the return type from the call to
DisplayAlert
. This method also returns a task and can be awaited. Await the call toDisplayAlert
. - Run the application, it will remain responsive while downloading search results.
- Compare the final solution to the start solution from Part 01. Notice only a few lines of code are different between the task-based async version and the original synchronous code.
Summary
In this exercise you used the task-based async pattern to download movie search results. You also compared the tasked-based async implementation with a synchronous version.