Duration
15 minutes
Goals
The primary goal of this lab is to modify an existing application to use the event-based async pattern to download search results from a web service.
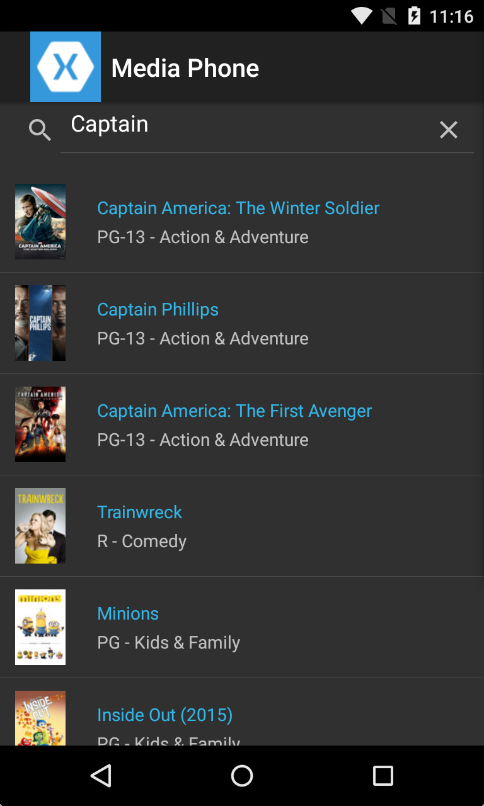
Required assets
The provided Exercise 1 folder contains a subfolder named Start with a solution you will use as starting point. There is also a Completed folder with a solution you can use to check your work. Please make sure you have these folders before you begin.
Challenge
Update an existing application to use the event-based async pattern when searching for movies.
- Examine the start solution.
- Add a new method to MovieApi.cs called SearchAsync that uses
WebClient
'sDownloadStringAsync
method and theDownloadStringCompleted
event
. - In MovieApi.cs, create a public
Action
named SearchComplete and invoke it when the search completes, passing in the movie data. - In SearchPage.xaml.cs, update the OnSearch method to use the new SearchAsync method and SearchComplete action.
Steps
Examine the Start Solution
- Open the MediaPhone shared project.
- Inspect SearchPage.xaml; it displays a
SearchBar
and aListView
. - Open the Data folder in the solution explorer. The code in MovieApi.cs is used to query the iTunes movie database. SearchResult.cs holds a model object class to hold the returned data.
- Run the application and try searching for a movie.
- Perform a search using a keyword that will return multiple results (try captain). Try to interact with the UI before the search results are returned? What happened?
Add an Asynchronous Search Method
- Open MovieApi.cs.
- Create a new
void
method named SearchAsync that accepts a singlestring
parameter named text. - Copy the null check code, the creation of the local query
string
and the creation of theWebClient
from the old Search method. - Instead of calling the
WebClient
'sDownloadString
method, subscribe to theDownloadStringCompleted
EventHandler
. You may use any subscription method you prefer; the completed solution uses a lambda expression. - Within the handler, check the
DownloadStringCompletedEventArgs
'sError
property fornull
. - If
Error
is null, we can assume the download completed successfully. Save theDownloadStringCompletedEventArgs
'sResult
property to a localstring
variable named resultText. - Parse the resultText using the static
JsonConvert.DeserializeObject
method and save to local variable named results. - Call the
WebClient
wc'sDownloadStringAsync
method. For the parameter, create a newUri
passing query into the constructor of theUri
.
Provide the Search Results
We'll create a public action that will provide the deserialized results to a subscriber when the results are ready.
- In MovieApi.cs, create a public
Action
that accepts anIEnumerable
ofSearchItem
s and anException
named SearchComplete. - Add the
event
keyword before theAction
declaration to limit its accessibility.
The deserialized results variable contains a property that is also named results which is a - If
e.Error
is not null, invoke the SearchCompleteAction
passing innull
for the first parameter and the error for the second parameter.
List
of SearchItem
s.
When invoking the Action
, pass in results.results as the first parameter and null
for 2nd Exception
parameter.
Invoke the Async Search method
- Open SearchPage.xaml.cs.
- In the OnSearch method, delete the
try
,catch
, andfinally
blocks. - Create a local instance of
MovieApi
named api. Subscribe to its SearchCompleteevent
that we created in the last step. - In the handler, check if the
Exception
exists, and if so return. - If the
Exception
isnull
, use aforeach
loop to add each item from the passed inIEnumerable
ofSearchItem
s to theListView
's data source named Data. - Set the searchProgress's IsRunning property to null to hide the activity indicator.
- After the handler, call api's SearchAsync method, passing in the search terms text from the searchBar.
- Run the application. Notice that the
ActivityIndicator
is now visible during searches.
Summary
In this exercise, you updated an existing application to use the event-based async pattern to query a web service for search results. This allowed the UI to remain responsive while waiting for the results from the web service.